Common Mistakes to Avoid When Creating a React Application
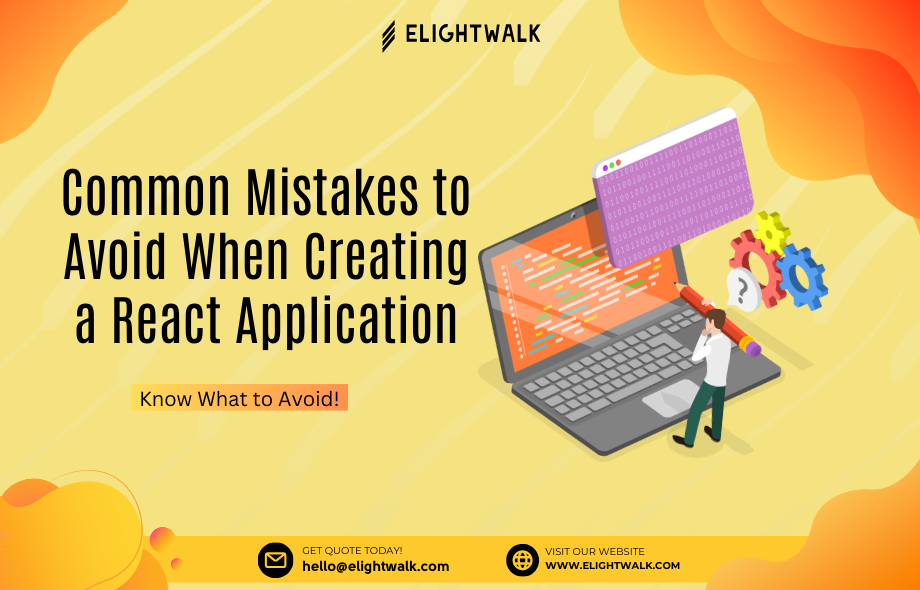
This guide seeks to help React developers guarantee strong and practical projects and avoid typical errors in creating React applications. It also shows how important it is to know React's basic rules, adhere to best practices, and write clean code that works well. Developers can improve their web development skills by avoiding these mistakes and creating dynamic and functional user interfaces.
What is ReactJS?
Facebook created the open-source JavaScript toolkit known as ReactJS. It is used to develop user interfaces for single-page applications. It focuses on building reusable components and efficiently updating and rendering the right parts of a web application using a virtual DOM.
Why ReactJS for front-end development?
ReactJS is widely used for front-end development because it simplifies building dynamic and responsive web interfaces. Its component-based architecture, virtual DOM, unidirectional data flow, and integration flexibility with other tools make it ideal for creating high-performance, scalable, and maintainable applications.
Not organizing the project structure properly.
Mistake: Placing all files in a single folder or not using modular structures leads to cluttered codebases.
Why It's a Problem: The more users the application has, the more complicated it is to administer and expand.
Solution:
- Adopt a clear folder structure that separates components, hooks, services, and assets into their respective directories.
Example structure:
src/
├── components/
├── hooks/
├── pages/
├── services/
├── styles/
└── utils/
Overusing or Underusing State
- Mistake:
- Overusing state, e.g., storing unnecessary data like derived values or redundant data in the state.
- Not properly use state, such as React's state management, for updating the user interface dynamically.
- Why It's a Problem: Overuse can cause unnecessary re-renders, while underuse leads to inflexible, hard-to-maintain components.
- Solution:
- Store only what's necessary in the state.
- Use derived values and memoization where applicable, e.g., useMemo and useCallback.
- Opt for state management libraries (e.g., Redux, Zustand, or Context API) for complex state requirements.
Ignoring Performance Optimization
- Mistake:
- Failing to optimize large lists or components, resulting in slow rendering.
- Using anonymous functions or inline functions unnecessarily in props.
- Why It's a Problem: Impacts app performance, especially in larger applications.
- Solution:
- Use tools like React. Memo to prevent unnecessary re-renders of components.
- Implement code-splitting and lazy loading with React. Lazy loading and Suspense.
- Optimize lists with libraries like react-window or react-virtualized.
Neglecting Error Boundaries
- Mistake: Failing to handle errors gracefully within components.
- Why It's a Problem: Uncaught errors can crash the entire app, leading to a poor user experience.
- Solution: Use error boundaries to catch errors in components and display fallback UI. Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError() {
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Hardcoding Values Instead of Using Props or State
- Mistake: Hardcoding values directly into components.
- Why It's a Problem: Limits reusability and makes the component inflexible.
- Solution: Pass dynamic values through props or manage them with state
Neglecting Dependency Arrays in Hooks
- Mistake: Forgetting to specify dependencies in hooks like useEffect.
- Why It's a Problem: It can cause infinite loops or unintended behavior.
- Solution: Always include dependencies in the dependency array to ensure the hook behaves as expected.
Direct DOM Manipulation
- Mistake: Direct DOM manipulation (e.g., document.querySelector) instead of React's ref system.
- Why It's a Problem: It can lead to inconsistencies and bypass React's rendering flow.
- Solution: Use useRef to access DOM elements.
Final Thoughts
Creating a React app can be pleasing and sometimes difficult. Achieving ideal performance, scalability, and maintainability is also challenging. Avoiding common mistakes will help React to achieve its best. These include overlooked performance optimizations, mismanaged states, and poor project structures.
You should also follow best practices, such as error boundaries and hooks. A well-structured, optimized, and error-resistant React js application improves users' experience and makes it easier to build and expand in the future. Keep learning, follow best practices, and embrace React. It will help you build robust, successful apps.
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- Games
- Gardening
- Health
- Home
- Literature
- Music
- Networking
- Other
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
- IT, Cloud, Software and Technology