How to Protect Laravel Apps Like a Pro - Security Secrets
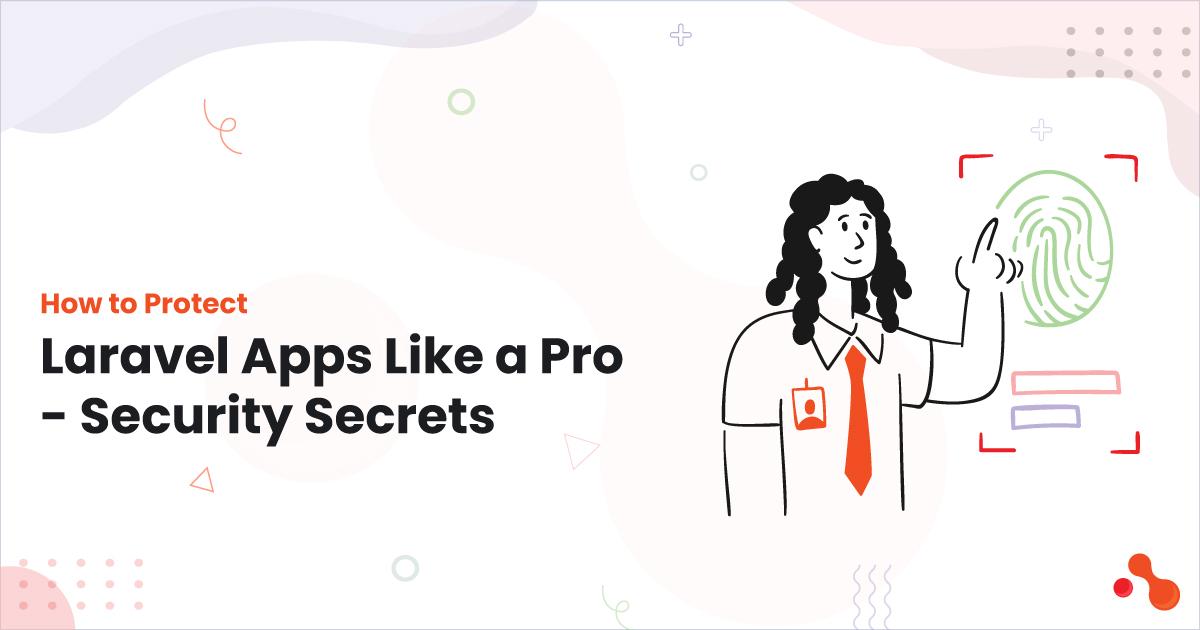
Introduction
Securing web applications is of paramount importance in today’s digital landscape, and Laravel, a popular PHP framework, provides robust features to help developers create secure applications. However, beyond the basic security measures, advanced practices are necessary to ensure comprehensive protection.
This article delves into advanced security measures to secure Laravel applications, covering areas such as authentication, authorization, data encryption, input validation, and more. Securing a Laravel application by implementing advanced security practices will ensure you protect your application from potential threats. However, it takes the skills of expert Laravel developers to achieve this feat.
Laravel 11, continuing its tradition of being a robust and secure PHP framework, introduces several advanced security features to help developers protect their applications against emerging threats. This article explores the advanced Laravel security features, highlighting how these features can be leveraged to build secure and resilient web applications.
Laravel Security
Laravel has built-in security features that protect against common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
However, relying solely on these features isn't enough. Advanced security measures are required to address more sophisticated attacks and ensure a secure environment for the application and its users.
A professional Laravel development company will have the expertise and knowledge to take advantage of all the security features that Laravel has to offer. In fact, it will work in your favor to hire a firm that is an official Laravel Partner, especially since they will have an advanced set of skills and appropriate resources.
Acquaint Softtech is a software development outsourcing company in India and also an official Laravel Partner. We have a dedicated team of Laravel developers with the skills and ability to implement basic Laravel security as well as the advanced Laravel security features.
Some of the basic security features include:
- Secure Your Environment
- HTTPS Everywhere
- Authentication and Authorization
- Database Security
- Input Validation and Sanitization
- CSRF Protection
- Security Headers
- Rate Limiting and Throttling
- Logging and Monitoring
- File Upload Security
- Configuration Security
- Regular Updates and Patching
- Security Testing
- Disaster Recovery Planning
Cybersecurity Secrets
Laravel is the ideal platform to develop state-of-the-art solutions for many reasons. It has several built-in security features, and with the release of version 11, it has become more secure. It has many secrets up its sleeve, making it the ideal choice for developing a secure solution.
Here are some of the cybersecurity secrets of Laravel:
- Multi-Factor Authentication (MFA): Implementing MFA adds an extra layer of security by requiring users to provide two or more verification factors to gain access. Laravel can integrate with MFA services like Google Authenticator and Authy or use custom implementations.
Password Security
- Hashing: Use Laravel's built-in bcrypt or argon2 hashing to store passwords securely.
- Password Policies: Enforce strong password policies, including minimum length, complexity, and periodic resets.
Secure APIs
- API Authentication: Use OAuth2 or JWT for secure API authentication.
- Rate Limiting: Implement rate limiting on API endpoints to prevent abuse.
- Input Validation: Validate and sanitize all API inputs to avoid injection attacks.
- SQL Injection Protection: Laravel uses Eloquent ORM, which utilizes prepared statements to prevent SQL injection attacks. Always use Eloquent or the query builder for database operations instead of raw SQL queries.
Improved Authentication Guarding:
- Enhanced multi-factor authentication (MFA) support.
- Improved API token management with expiration and revocation capabilities.
Advanced Encryption:
- Enhanced support for modern encryption algorithms.
- Simplified API for encrypting and decrypting data.
- Built-in support for encryption key rotation.
Enhanced Authorization:
- More granular authorization policies.
- Improved gate and policy functionalities to control user actions more precisely.
Comprehensive Validation:
- Expanded set of validation rules to handle more complex input scenarios.
- Improved custom validation rules for more robust input sanitization.
- CSRF Protection: Improved cross-site request forgery (CSRF) protection with enhanced token generation and validation mechanisms.
Enhanced Session Security:
- Secure session management with support for same-site cookies.
- Improved session handling to prevent session fixation attacks.
Improved Logging and Monitoring:
- Built-in support for advanced logging mechanisms to detect and respond to security incidents.
- Integration with modern monitoring tools for real-time security alerts.
Database Security:
- Improved database query sanitization to prevent SQL injection.
- Enhanced support for parameterized queries.
- Package Integrity Verification: Enhanced Composer integration is used to verify package integrity and detect compromised dependencies.
Dependency Management:
- Automated checks for vulnerable dependencies.
- Built-in tools for managing and updating dependencies securely.
- Security Middleware: New and improved middleware for handling common security tasks like XSS protection, content security policy (CSP) enforcement, and more.
API Security
- OAuth2 and JWT Authentication: Enhanced support for OAuth2 and JWT (JSON Web Tokens) for secure API authentication.
- Rate Limiting for APIs: Advanced rate limiting and throttling mechanisms for API endpoints to protect against abuse.
Testing Application Security
Ensuring the security of a Laravel application involves a comprehensive approach to testing. Here are some essential tips to help you test the security of your Laravel application effectively:
Use Automated Security Tools:
- Laravel Security Checker: Utilize tools like Laravel Security Checker to scan your application for known vulnerabilities in your dependencies.
- Static Analysis Tools: Use tools like PHPStan or Psalm to analyze your code for potential security issues.
Conduct Regular Penetration Testing:
- Hire Security Experts: Engage professional penetration testers to find vulnerabilities that automated tools might miss.
- Simulate Attacks: Conduct simulated attacks on your application to test its resilience against common threats such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Perform Code Reviews:
- Peer Reviews: Implement regular code reviews by peers to catch security issues early in the development process.
- Static Code Analysis: Use static code analysis tools to review your code for security vulnerabilities automatically.
- Implement Unit and Integration Tests:
- Security-Specific Tests: Write unit and integration tests to check for security vulnerabilities, such as unauthorized access or data leakage.
- Test Coverage: Ensure your tests cover all critical parts of your application, including authentication, authorization, and data validation.
Validate Input Thoroughly:
- Sanitize User Input: Always sanitize and validate all user inputs to prevent SQL injection and XSS attacks.
- Use Laravel Validation: Leverage Laravel’s built-in validation mechanisms to enforce data integrity and security.
Check Authentication and Authorization:
- Test Authentication Flows: Verify that all authentication mechanisms are robust and secure.
- Check Authorization Rules: Ensure that access control rules are correctly implemented and enforced.
Monitor and Log Activities:
- Implement Logging: Use Laravel’s logging features to keep track of application activities and potential security breaches.
- Monitor Logs: Regularly monitor and analyze logs for unusual or suspicious activities.
Test Error Handling:
- Graceful Error Handling: Ensure your application handles errors gracefully without revealing sensitive information.
- Custom Error Pages: Implement custom error pages to prevent information leakage through default error messages.
Secure Configuration:
- Environment Variables: Secure sensitive configuration data using environment variables.
- Configuration Management: Regularly review and update your application’s configuration settings to ensure they are secure.
Update Dependencies Regularly:
- Composer Updates: Regularly update your Composer dependencies to ensure you have the latest security patches.
- Monitor Vulnerabilities: Stay informed about vulnerabilities in the libraries and packages you use.
Use HTTPS:
- SSL/TLS Certificates: Ensure your application uses HTTPS to encrypt data transmitted between the client and server.
- Enforce HTTPS: Use middleware to enforce HTTPS for all requests.
Perform Security Audits:
- Regular Audits: Conduct regular security audits to assess the overall security posture of your application.
- Audit Tools: Auditing tools are used to automate parts of the security assessment process.
Implement Rate Limiting:
- Throttling Middleware: Use Laravel’s throttling middleware to prevent brute force attacks by limiting the number of requests a user can make in a given time frame.
Protect Against CSRF:
- CSRF Tokens: Ensure all forms and state-changing requests include CSRF tokens to protect against cross-site request forgery attacks.
- Verify CSRF Tokens: Use Laravel’s built-in CSRF protection mechanisms to verify tokens on incoming requests.
Regularly Backup Data:
- Automated Backups: Set up automated backups of your database and critical data to recover quickly in case of a security breach.
- Backup Security: Ensure that backups are securely stored and encrypted.
Implementing these tips can enhance the security of your Laravel application and protect it from various cyber threats. Regular testing and proactive security measures are crucial in maintaining a secure application environment.
Hire Laravel Developers
Hire Laravel developers from a professional firm like Acquaint Softtech. This is crucial for ensuring the security and success of your web applications.
Here are some key benefits:
Expertise in Security Best Practices:
- Knowledge of Security Features: Businesses benefit from professional Laravel developers knowledge of security. They are well-versed in the framework’s built-in security features, such as CSRF protection, SQL injection prevention, and encryption.
- Regular Updates: They stay updated with the latest security best practices and vulnerabilities, protecting your application against emerging threats.
Customized Security Solutions:
- Tailored Security Measures: Experienced developers can implement customized security measures tailored to your application needs, enhancing overall security.
- Risk Mitigation: They can identify potential security risks unique to your application and develop strategies to mitigate them effectively.
Efficient Code Management:
- Clean and Maintainable Code: Professional developers write clean, maintainable, and secure code, reducing the risk of security vulnerabilities caused by poor coding practices.
- Code Reviews and Audits: They conduct regular code reviews and security audits to ensure the codebase remains secure over time.
Advanced Security Implementations:
- Data Encryption: Implement advanced data encryption techniques to protect sensitive information.
- Authentication and Authorization: Set up robust authentication and authorization mechanisms to control access to your application.
Compliance with Standards:
- Regulatory Compliance: Professional developers ensure that your application complies with relevant security standards and regulations, such as GDPR, HIPAA, and PCI-DSS.
- Security Certifications: They often hold security certifications and follow industry standards, providing an added layer of assurance.
Proactive Threat Detection and Prevention:
- Regular Monitoring: Implement continuous monitoring and logging to detect and respond to security threats in real time.
- Security Patches: Apply security patches and updates promptly to protect against known vulnerabilities.
Efficient Use of Security Tools:
- Automated Tools: Utilize automated security tools and frameworks to identify and fix vulnerabilities quickly.
- Static and Dynamic Analysis: Perform static and dynamic security analysis to detect potential security issues early in development.
Enhanced Performance and Scalability:
- Optimized Security: Optimize security measures without compromising the performance and scalability of your application.
- Efficient Resource Management: Manage resources to handle security tasks effectively, ensuring your application remains performant.
Focus on Core Business Activities:
- Delegation of Security Tasks: By hiring professional developers, you can delegate security tasks to experts and focus on your core business activities.
- Peace of Mind: You can rest assured that your application's security is in the hands of skilled professionals.
Long-term Security Strategy:
- Ongoing Support: Professional developers provide continuing support and maintenance, ensuring your application remains secure over its lifecycle.
- Strategic Planning: Develop long-term security strategies to adapt to evolving threats and ensure continuous protection.
When you hire remote developers from Acquaint Softtech, you can ensure your web applications are secure and compliant and resilient against various cyber threats. Our expertise and proactive approach to security will help you build robust and reliable applications, providing a safe environment for your users and business.
Conclusion
Securing a Laravel application requires a multifaceted approach. it includes addressing various aspects, from authentication and data encryption to server security and continuous monitoring. Implementing these advanced security measures helps protect against sophisticated threats and ensures the safety of your application and its users.
Regularly updating and auditing your security practices is crucial to staying ahead of potential vulnerabilities.
It's a continuous process that requires vigilance and regular updates to stay ahead of potential threats.
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- Games
- Gardening
- Health
- Home
- Literature
- Music
- Networking
- Other
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
- IT, Cloud, Software and Technology